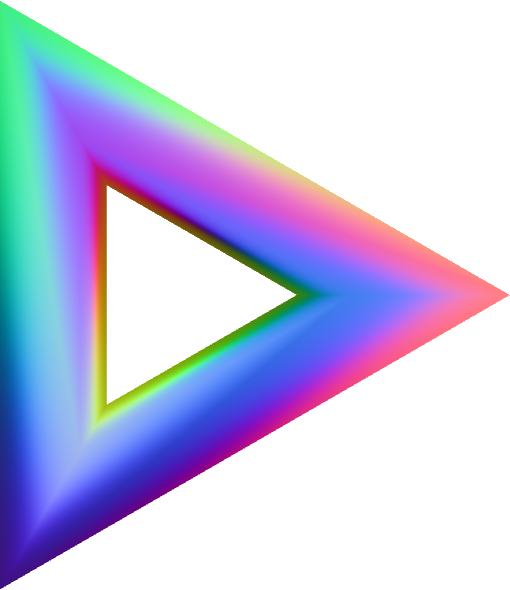
Threeasy
v0.1.20
Threeasy Components
ThreeJS has no prescribed method of organising components. As a result, most
demos dont use components. This makes most complicated demos very hard to
read, because everything is competing with scope in one file. Threeasy has a
simple but opinionated Component
. It encourages splitting functionality
into separeate files. This is how coding in the real world works. The
Threeasy Component
is a class. In the constructor
you can set the basic
state of an object. It also exposes an animate()
function that is
automatically hooked up to the render loop for you. Here is the most basic
setup:
import * as THREE from "three";
import ThreeasyComponent from "threeasy/component.js";
export default class Box extends ThreeasyComponent {
constructor(app) {
super(app);
// setup
}
animate() {
// animate
}
}
In App.js when you want to use the Component
, you must pass the app
to
it.
import myComponent from '/myComponent.js';
const instance = new myComponent(app);
Example In this example a Box is created and animated. By storing the Box
in its own file, things are much more legible, and easier for a newcomer to understand.
Threeasy Components
// APP.JS
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
import Threeasy from "threeasy";
import Box from "./box.js";
const app = new Threeasy(THREE);
app.scene.background = new THREE.Color("dodgerblue");
app.camera.position.z = 15;
new OrbitControls(app.camera, app.renderer.domElement);
const boxes = [];
for (let i = 0; i < 20; i++) {
boxes.push(new Box(app));
}
// BOX.JS
import * as THREE from "three";
import ThreeasyComponent from "threeasyComponent";
export default class Box extends ThreeasyComponent {
constructor(app) {
super(app);
this.mat = new THREE.MeshNormalMaterial();
this.geo = new THREE.BoxGeometry();
this.mesh = new THREE.Mesh(this.geo, this.mat);
this.mesh.position.set(
this.getRandomVal(),
this.getRandomVal(),
this.getRandomVal()
);
this.dir = {
x: this.getRandomVal() / 500,
y: this.getRandomVal() / 500,
};
this.app.scene.add(this.mesh);
}
getRandomVal() {
return Math.random() * 20 - 10;
}
animate() {
this.mesh.rotation.x += this.dir.x;
this.mesh.rotation.y += this.dir.y;
}
}
See example