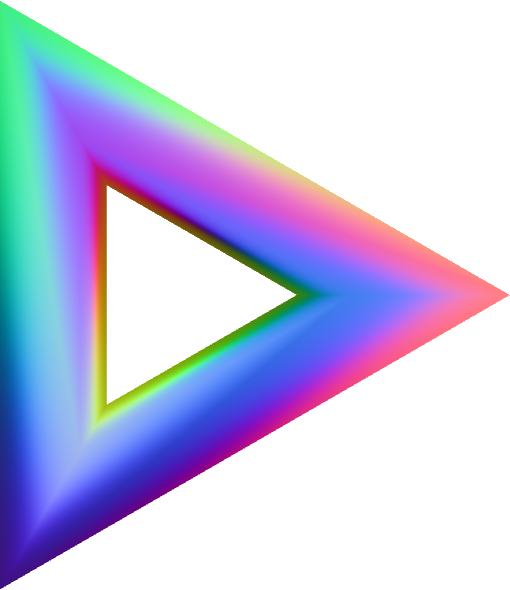
Threeasy
v0.1.20
Inline Animations
Most ThreeJS projects have an animation loop. These can get really
complicated for a beginner, and fast. Typically there are about 20-30 lines
of setup code that distract the user from the actual animation. Also, the
code to create your object, and the code to animate it are in different
places. The new user needs to remember where the code related to their
object is! There is also no convention around naming the animation function.
Is it animate
, render
, loop
, raf
? Threeasy gets around this by
letting you simply declare inline animations, beside the mesh or object that
you’re working on using app.animator.add()
. app.animator.add()
maintains
an array of all of your animations, ready to execute them on every frame.
Basic Threeasy Animation
import * as THREE from "three";
import Threeasy from "threeasy";
const app = new Threeasy(THREE);
const mat = new THREE.MeshBasicMaterial({ color: "white" });
const geo = new THREE.BoxGeometry();
const mesh = new THREE.Mesh(geo, mat);
app.animator.add(() => (mesh.rotation.y += 0.01));
app.scene.add(mesh);
See example