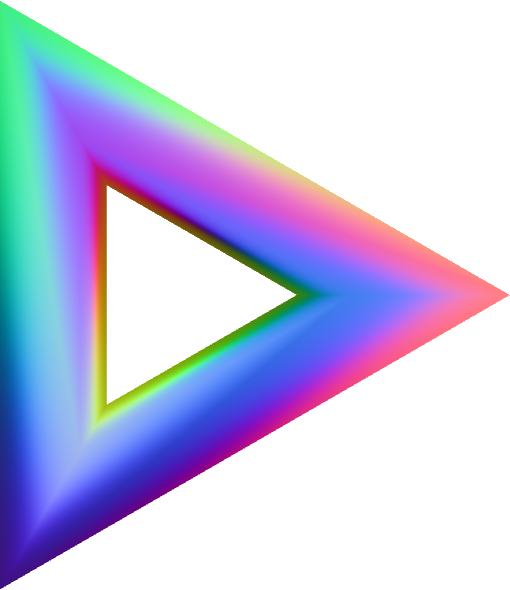
Threeasy
v0.1.20
Changing Renderer
Threeasy automatically sets up the default THREE Renderer
for you. But
you’re not limited to that, you can simply change it. To update the function
that is used to render the scene, you can pass a new function to
app.render
.
Change renderer
import * as THREE from "three";
import * as THREE from "three";
import { EffectComposer } from "three/addons/postprocessing/EffectComposer.js";
import { RenderPass } from "three/addons/postprocessing/RenderPass.js";
import { ShaderPass } from "three/addons/postprocessing/ShaderPass.js";
import { DotScreenShader } from "three/addons/shaders/DotScreenShader.js";
import Threeasy from "threeasy";
const app = new Threeasy(THREE);
app.camera.position.set(0, 2, 2);
app.camera.lookAt(0, 0, 0);
const mat = new THREE.MeshNormalMaterial();
const geo = new THREE.BoxGeometry();
const mesh = new THREE.Mesh(geo, mat);
const composer = new EffectComposer(app.renderer);
composer.addPass(new RenderPass(app.scene, app.camera));
const effect1 = new ShaderPass(DotScreenShader);
effect1.uniforms["scale"].value = 4;
composer.addPass(effect1);
// THIS RESETS THE RENDERER AND ADDS IT TO THE RENDER LOOP
app.render = () => composer.render();
app.animate(() => {
mesh.rotation.y += 0.01;
});
app.scene.add(mesh);
See example