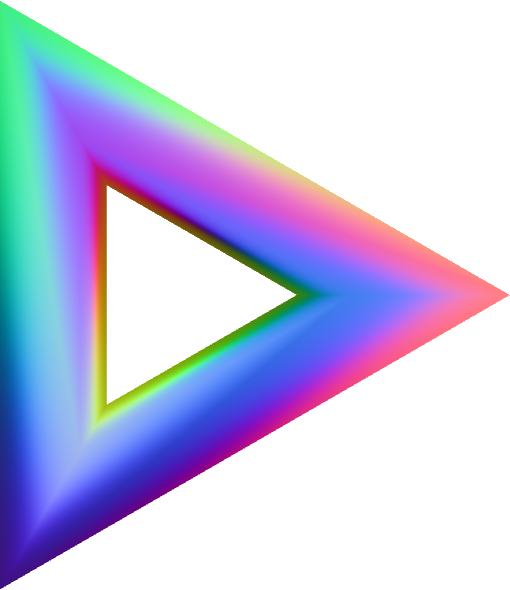
Threeasy
v0.1.20
Interactions
Often you’ll want to add some interactivity to your scene. Threeasy provides
a few helper functions to make this easier. Interactions can be expensive,
so by default Threeasy doesnt enable them. To enable them, you need to pass
the interactions: true
as an option to the Threeasy constructor. All
interations will return two variables. The javascript event
, and the
Three.js object
that was interacted with. ## Click To add a click, we need
to add two things to the interactions manager. First, the object we want to
listen to. In this case, we’ll use a cube. Second, the callback function.
This function will be called when the object is clicked.
Threeasy Interactions - Click
import * as THREE from "three";
import Threeasy from "threeasy";
const app = new Threeasy(THREE, { interactions: true });
const box = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(box, material);
let color = 0;
const colors = [
new THREE.Color(0x00ff00),
new THREE.Color(0xff0000),
new THREE.Color(0x0000ff),
];
// add a click listener to the cube
app.interactions.onClick(cube, (e) => {
color = (color + 1) % colors.length;
cube.material.color = colors[color];
});
app.scene.add(cube);
See example
Hover To add hover events we also add the object and a callback function.
However, this time we pass an object with two functions, enter and leave.
Threeasy Interactions - Hover
import * as THREE from "three";
import Threeasy from "threeasy";
const app = new Threeasy(THREE, { interactions: true });
const box = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(box, material);
app.interactions.onHover(cube, {
// enter event
enter: (e) => {
cube.material.color = new THREE.Color(0xff00ff);
},
// leave event
leave: (e) => {
cube.material.color = new THREE.Color(0xffff00);
},
});
app.scene.add(cube);
See example